 |
Script Coder |
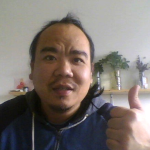 |
Joined: May 07, 2014 Posts: 3984 Location: Canada
|
Sample full animation effect created by this plug-in: 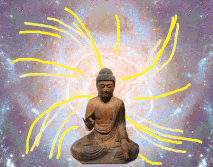 Cropped sample: 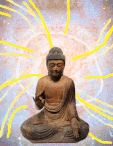 This plug-in will rotate all your linked layers. 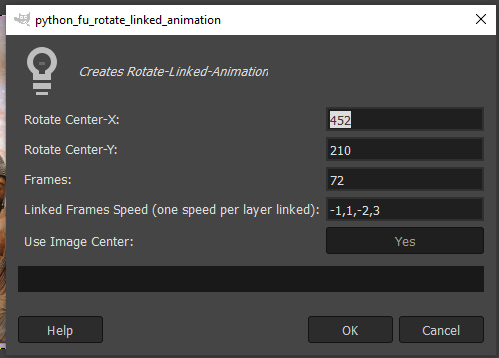 Linked frame speed, is a string of numbers (comma separated) that tell the plug-in how to rotate the linked frames. In example shown above, -1,1,-2,3 will tell it to rotate first linked layer at negative full speed (full revolution) rotate 2nd linked layer at full speed rotate 3rd linked layer at negative double speed rotate 4th linked layer at 3x speed. code below. #!/usr/bin/env python # rotate-linked-animation.py # Created by TT # # License: GPLv3 # This program is free software: you can redistribute it and/or modify # it under the terms of the GNU General Public License as published by # the Free Software Foundation, either version 3 of the License, or # (at your option) any later version. # # This program is distributed in the hope that it will be useful, # but WITHOUT ANY WARRANTY# without even the implied warranty of # MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the # GNU General Public License for more details. # # To view a copy of the GNU General Public License # visit: http://www.gnu.org/licenses/gpl.html # # # ------------ #| Change Log | # ------------ # Rel 1: Initial release # Rel 2: Leave the visibility unchanged of linked layers, added "Change Opacity" and "Move Layers" toggles as suggested by PixLab http://gimpchat.com/viewtopic.php?p=284716#p284716 from gimpfu import * import random import math
def ease_in_out(t): """Ease-in-out function: acceleration until halfway, then deceleration""" return 2*t*t if t < 0.5 else 1 - pow(-2*t + 2, 2) / 2 def python_rotate_linked_animation(image, layer, cx,cy,frames,speed,imgcenter): # pdb.gimp_image_undo_group_start(image) # pdb.gimp_context_push() #YOUR CODE BEGINS======================= imgcenter = int(imgcenter) if (imgcenter==1): cx = image.width/2 cy = image.height/2 frames = int(frames) new_image = pdb.gimp_image_new(image.width,image.height,RGB) pdb.gimp_display_new(new_image) #get linked layers and reverse the order linkedlayers = [] visible = [] positions = [] for l in image.layers: if pdb.gimp_item_get_linked(l) == TRUE: position = pdb.gimp_image_get_item_position(image,l) positions.append(position) linkedlayers.append(l) visible.append(pdb.gimp_item_get_visible(l)) linkedlayers.reverse() visible.reverse() positions.reverse()
speed = speed.split(",") speed.reverse()
#hide all linked layers so it doesn't affect our animation layers for l in linkedlayers: pdb.gimp_item_set_visible(l,FALSE) angle = 360.0/frames/360.0*math.pi*2.0 for j in range(0,frames): toberemoved = [] for i in range(0,len(linkedlayers)): linkedlayer = linkedlayers[i] new_layer = pdb.gimp_layer_new_from_drawable(linkedlayer,image) pdb.gimp_item_set_linked(new_layer,FALSE) pdb.gimp_image_insert_layer(image,new_layer,None,positions[i]) pdb.gimp_item_set_visible(new_layer,TRUE) new_layer = pdb.gimp_item_transform_rotate(new_layer,angle*j*float(speed[i]),FALSE,cx,cy) toberemoved.append(new_layer) visible_layer = pdb.gimp_layer_new_from_visible(image,new_image,'frame') pdb.gimp_image_insert_layer(new_image,visible_layer,None,0)
for l in toberemoved: pdb.gimp_image_remove_layer(image,l)
#set it back to whatever visibility it was for i in range(0,len(linkedlayers)): l = linkedlayers[i] v = visible[i] pdb.gimp_item_set_visible(l,v) #YOUR CODE ENDS ======================== # pdb.gimp_context_pop() # pdb.gimp_image_undo_group_end(image) pdb.gimp_displays_flush()
#return
register( "python_fu_rotate_linked_animation", "Creates Rotate-Linked-Animation", "Creates Rotate-Linked-Animation", "TT", "TT", "October 30, 2023", "<Image>/Python-Fu/Rotate-Linked-Animation...", "*", # Create a new image, don't work on an existing one [ #INPUT BEGINS (PF_INT, "cx", "Rotate Center-X:", 452), (PF_INT, "cy", "Rotate Center-Y:", 210), (PF_INT, "frames", "Frames:", 72), #1.5 to 2 seconds (PF_STRING, "speed", "Linked Frames Speed (one speed per layer linked):", "-1,1,-2,3"), # alias PF_VALUE (PF_TOGGLE, "imgcenter", "Use Image Center:", 1), #INPUT ENDS ], [], python_rotate_linked_animation)
main()
# Below is all the example input types for INPUTS for the plug-in which can be cut and pasted into #INPUT BEGINS section and edited to taste # (PF_INT, "p0", "_INT:", 0), # PF_INT8, PF_INT16, PF_INT32 similar but no difference in Python. # (PF_FLOAT, "p02", "_FLOAT:", 3.141), # (PF_STRING, "p03", "_STRING:", "foo"), # alias PF_VALUE # (PF_TEXT, "p04", "TEXT:", "bar"), # # PF_VALUE # # Pick one from set of choices # (PF_OPTION,"p1", "OPTION:", 0, ["0th","1st","2nd"]), # initially 0th is choice # (PF_RADIO, "p16", "RADIO:", 0, (("0th", 1),("1st",0))), # note bool indicates initial setting of buttons # # PF_RADIO is usually called a radio button group. # # SLIDER, ADJUSTMENT types require the extra parameter of the form (min, max, step). # (PF_TOGGLE, "p2", "TOGGLE:", 1), # initially True, checked. Alias PF_BOOL # # PF_TOGGLE is usually called a checkbox. # (PF_SLIDER, "p3", "SLIDER:", 0, (0, 100, 10)), # (PF_SPINNER, "p4", "SPINNER:", 21, (1, 1000, 50)), # alias PF_ADJUSTMENT # # Pickers ie combo boxes ie choosers from lists of existing Gimp objects # (PF_COLOR, "p14", "_COLOR:", (100, 21, 40) ), # extra param is RGB triple # # PF_COLOUR is an alias by aussie PyGimp author lol # (PF_IMAGE, "p15", "IMAGE:", None), # should be type gimp.image, but None works # (PF_FONT, "p17", "FONT:", 0), # (PF_FILE, "p18", "FILE:", 0), # (PF_BRUSH, "p19", "BRUSH:", 0), # (PF_PATTERN, "p20", "PATTERN:", 0), # (PF_GRADIENT, "p21", "GRADIENT:", 0), # (PF_PALETTE, "p22", "PALETTE:", 0), # (PF_LAYER, "p23", "LAYER:", None), # (PF_CHANNEL, "p24", "CHANNEL:", None), # ??? Usually empty, I don't know why. # (PF_DRAWABLE, "p25", "DRAWABLE:", None), # # Mostly undocumented, but work # (PF_VECTORS, "p26", "VECTORS:", None), # (PF_FILENAME, "p27", "FILENAME:", 0), # (PF_DIRNAME, "p28", "DIRNAME:", 0) # # PF_REGION might work but probably of little use. See gimpfu.py.
Attribution for images used to create the .xcf https://en.wikipedia.org/wiki/File:M101 ... 06-10a.jpghttps://commons.wikimedia.org/wiki/File ... _(Amitabha),_Kamakura_period,_12th-13th_century,_wood_with_gold_leaf_and_inlaid_crystal_eyes_-_Tokyo_National_Museum_-_DSC05345.JPG
Attachments: |
File comment: the .xcf that I used to run to create sample animation
buddha.xcf [5.02 MiB]
Downloaded 28 times
|
_________________ TinT
|
|