 |
GimpChat Member |
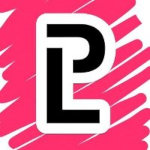 |
Joined: May 24, 2021 Posts: 785 Location: SEA - South East Asia
|
trandoductin wrote: PixLab wrote: trandoductin wrote: version 7 (50% of the time move the copy down, 50% of the time move the original character down to get more variations).
Maybe as an option to tick? for people who want consistency or re-do the pattern for some reason like bigger or whatever and not getting a random luck  There's lots of randomness already, that redoing won't produce same pattern. Oh... indeed, you're right I did change line 208 in your latest (203 in V6) just to have the html name and the hexa name as layer's name instead of the repetitive "text pattern #x" new_layer = pdb.gimp_layer_new(image,image.width,layer_copy.height,RGBA_IMAGE,colors[c][1]+" / "+colors[c][2],100,LAYER_MODE_NORMAL)
When I saw that you have inputted all those html names > I was too sad to not use them  Attachment:
screenshot_20230309-194322.jpg [ 198.79 KiB | Viewed 1451 times ]
EDIT: 120 was not enough Yeah... OK, 650+ it's a bit over the top colors = [ ["1","Black (W3C)","#000000"], ["2","Night","#0C090A"], ["3","Charcoal","#34282C"], ["4","Oil","#3B3131"], ["5","Dark Gray","#3A3B3C"], ["6","Light Black","#454545"], ["7","Black Cat","#413839"], ["8","Iridium","#3D3C3A"], ["9","Black Eel","#463E3F"], ["10","Black Cow","#4C4646"], ["11","Gray Wolf","#504A4B"], ["12","Vampire Gray","#565051"], ["13","Iron Gray","#52595D"], ["14","Gray Dolphin","#5C5858"], ["15","Carbon Gray","#625D5D"], ["16","Ash Gray","#666362"], ["17","DimGray or DimGrey (W3C)","#696969"], ["18","Nardo Gray","#686A6C"], ["19","Cloudy Gray","#6D6968"], ["20","Smokey Gray","#726E6D"], ["21","Alien Gray","#736F6E"], ["22","Sonic Silver","#757575"], ["23","Platinum Gray","#797979"], ["24","Granite","#837E7C"], ["25","Gray or Grey (W3C)","#808080"], ["26","Battleship Gray","#848482"], ["27","Gunmetal Gray","#8D918D"], ["28","DarkGray or DarkGrey (W3C)","#A9A9A9"], ["29","Gray Cloud","#B6B6B4"], ["30","Silver (W3C)","#C0C0C0"], ["31","Pale Silver","#C9C0BB"], ["32","Gray Goose","#D1D0CE"], ["33","Platinum Silver","#CECECE"], ["34","LightGray or LightGrey (W3C)","#D3D3D3"], ["35","Silver White","#DADBDD"], ["36","Gainsboro (W3C)","#DCDCDC"], ["37","Platinum","#E5E4E2"], ["38","Metallic Silver","#BCC6CC"], ["39","Blue Gray","#98AFC7"], ["40","Roman Silver","#838996"], ["41","LightSlateGray or LightSlateGrey (W3C)","#778899"], ["42","SlateGray or SlateGrey (W3C)","#708090"], ["43","Rat Gray","#6D7B8D"], ["44","Slate Granite Gray","#657383"], ["45","Jet Gray","#616D7E"], ["46","Mist Blue","#646D7E"], ["47","Marble Blue","#566D7E"], ["48","Slate Blue Grey","#737CA1"], ["49","Light Purple Blue","#728FCE"], ["50","Azure Blue","#4863A0"], ["51","Blue Jay","#2B547E"], ["52","Charcoal Blue","#36454F"], ["53","Dark Blue Grey","#29465B"], ["54","Dark Slate","#2B3856"], ["55","Deep-Sea Blue","#123456"], ["56","Night Blue","#151B54"], ["57","MidnightBlue (W3C)","#191970"], ["58","Navy (W3C)","#000080"], ["59","Denim Dark Blue","#151B8D"], ["60","DarkBlue (W3C)","#00008B"], ["61","Lapis Blue","#15317E"], ["62","New Midnight Blue","#0000A0"], ["63","Earth Blue","#0000A5"], ["64","Cobalt Blue","#0020C2"], ["65","MediumBlue (W3C)","#0000CD"], ["66","Blueberry Blue","#0041C2"], ["67","Canary Blue","#2916F5"], ["68","Blue (W3C)","#0000FF"], ["69","Samco Blue","#0002FF"], ["70","Bright Blue","#0909FF"], ["71","Blue Orchid","#1F45FC"], ["72","Sapphire Blue","#2554C7"], ["73","Blue Eyes","#1569C7"], ["74","Bright Navy Blue","#1974D2"], ["75","Balloon Blue","#2B60DE"], ["76","RoyalBlue (W3C)","#4169E1"], ["77","Ocean Blue","#2B65EC"], ["78","Blue Ribbon","#306EFF"], ["79","Blue Dress","#157DEC"], ["80","Neon Blue","#1589FF"], ["81","DodgerBlue (W3C)","#1E90FF"], ["82","Glacial Blue Ice","#368BC1"], ["83","SteelBlue (W3C)","#4682B4"], ["84","Silk Blue","#488AC7"], ["85","Windows Blue","#357EC7"], ["86","Blue Ivy","#3090C7"], ["87","Blue Koi","#659EC7"], ["88","Columbia Blue","#87AFC7"], ["89","Baby Blue","#95B9C7"], ["90","CornflowerBlue (W3C)","#6495ED"], ["91","Sky Blue Dress","#6698FF"], ["92","Iceberg","#56A5EC"], ["93","Butterfly Blue","#38ACEC"], ["94","DeepSkyBlue (W3C)","#00BFFF"], ["95","Midday Blue","#3BB9FF"], ["96","Crystal Blue","#5CB3FF"], ["97","Denim Blue","#79BAEC"], ["98","Day Sky Blue","#82CAFF"], ["99","LightSkyBlue (W3C)","#87CEFA"], ["100","SkyBlue (W3C)","#87CEEB"], ["101","Jeans Blue","#A0CFEC"], ["102","Blue Angel","#B7CEEC"], ["103","Pastel Blue","#B4CFEC"], ["104","Light Day Blue","#ADDFFF"], ["105","Sea Blue","#C2DFFF"], ["106","Heavenly Blue","#C6DEFF"], ["107","Robin Egg Blue","#BDEDFF"], ["108","PowderBlue (W3C)","#B0E0E6"], ["109","Coral Blue","#AFDCEC"], ["110","LightBlue (W3C)","#ADD8E6"], ["111","LightSteelBlue (W3C)","#B0CFDE"], ["112","Gulf Blue","#C9DFEC"], ["113","Pastel Light Blue","#D5D6EA"], ["114","Lavender Blue","#E3E4FA"], ["115","White Blue","#DBE9FA"], ["116","Lavender (W3C)","#E6E6FA"], ["117","Water","#EBF4FA"], ["118","AliceBlue (W3C)","#F0F8FF"], ["119","GhostWhite (W3C)","#F8F8FF"], ["120","Azure (W3C)","#F0FFFF"], ["121","LightCyan (W3C)","#E0FFFF"], ["122","Light Slate","#CCFFFF"], ["123","Electric Blue","#9AFEFF"], ["124","Tron Blue","#7DFDFE"], ["125","Blue Zircon","#57FEFF"], ["126","Aqua or Cyan (W3C)","#00FFFF"], ["127","Bright Cyan","#0AFFFF"], ["128","Celeste","#50EBEC"], ["129","Blue Diamond","#4EE2EC"], ["130","Bright Turquoise","#16E2F5"], ["131","Blue Lagoon","#8EEBEC"], ["132","PaleTurquoise (W3C)","#AFEEEE"], ["133","Pale Blue Lily","#CFECEC"], ["134","Light Teal","#B3D9D9"], ["135","Tiffany Blue","#81D8D0"], ["136","Blue Hosta","#77BFC7"], ["137","Cyan Opaque","#92C7C7"], ["138","Northern Lights Blue","#78C7C7"], ["139","Blue Green","#7BCCB5"], ["140","MediumAquaMarine (W3C)","#66CDAA"], ["141","Magic Mint","#AAF0D1"], ["142","Light Aquamarine","#93FFE8"], ["143","Aquamarine (W3C)","#7FFFD4"], ["144","Bright Teal","#01F9C6"], ["145","Turquoise (W3C)","#40E0D0"], ["146","MediumTurquoise (W3C)","#48D1CC"], ["147","Deep Turquoise","#48CCCD"], ["148","Jellyfish","#46C7C7"], ["149","Blue Turquoise","#43C6DB"], ["150","DarkTurquoise (W3C)","#00CED1"], ["151","Macaw Blue Green","#43BFC7"], ["152","LightSeaGreen (W3C)","#20B2AA"], ["153","Seafoam Green","#3EA99F"], ["154","CadetBlue (W3C)","#5F9EA0"], ["155","Deep-Sea","#3B9C9C"], ["156","DarkCyan (W3C)","#008B8B"], ["157","Teal Green","#00827F"], ["158","Teal (W3C)","#008080"], ["159","Teal Blue","#007C80"], ["160","Medium Teal","#045F5F"], ["161","Dark Teal","#045D5D"], ["162","Deep Teal","#033E3E"], ["163","DarkSlateGray or DarkSlateGrey (W3C)","#25383C"], ["164","Gunmetal","#2C3539"], ["165","Blue Moss Green","#3C565B"], ["166","Beetle Green","#4C787E"], ["167","Grayish Turquoise","#5E7D7E"], ["168","Greenish Blue","#307D7E"], ["169","Aquamarine Stone","#348781"], ["170","Sea Turtle Green","#438D80"], ["171","Dull-Sea Green","#4E8975"], ["172","Dark Green Blue","#1F6357"], ["173","Deep-Sea Green","#306754"], ["174","Bottle Green","#006A4E"], ["175","SeaGreen (W3C)","#2E8B57"], ["176","Elf Green","#1B8A6B"], ["177","Dark Mint","#31906E"], ["178","Jade","#00A36C"], ["179","Earth Green","#34A56F"], ["180","Chrome Green","#1AA260"], ["181","Emerald","#50C878"], ["182","Mint","#3EB489"], ["183","MediumSeaGreen (W3C)","#3CB371"], ["184","Metallic Green","#7C9D8E"], ["185","Camouflage Green","#78866B"], ["186","Sage Green","#848B79"], ["187","Hazel Green","#617C58"], ["188","Venom Green","#728C00"], ["189","OliveDrab (W3C)","#6B8E23"], ["190","Olive (W3C)","#808000"], ["191","DarkOliveGreen (W3C)","#556B2F"], ["192","Military Green","#4E5B31"], ["193","Green Leaves","#3A5F0B"], ["194","Army Green","#4B5320"], ["195","Fern Green","#667C26"], ["196","Fall Forest Green","#4E9258"], ["197","Irish Green","#08A04B"], ["198","Pine Green","#387C44"], ["199","Medium Forest Green","#347235"], ["200","Jungle Green","#347C2C"], ["201","Cactus Green","#227442"], ["202","ForestGreen (W3C)","#228B22"], ["203","Green (W3C)","#008000"], ["204","DarkGreen (W3C)","#006400"], ["205","Deep Green","#056608"], ["206","Deep Emerald Green","#046307"], ["207","Hunter Green","#355E3B"], ["208","Dark Forest Green","#254117"], ["209","Lotus Green","#004225"], ["210","Seaweed Green","#437C17"], ["211","Shamrock Green","#347C17"], ["212","Green Onion","#6AA121"], ["213","Moss Green","#8A9A5B"], ["214","Grass Green","#3F9B0B"], ["215","Green Pepper","#4AA02C"], ["216","Dark Lime Green","#41A317"], ["217","Parrot Green","#12AD2B"], ["218","Clover Green","#3EA055"], ["219","Dinosaur Green","#73A16C"], ["220","Green Snake","#6CBB3C"], ["221","Alien Green","#6CC417"], ["222","Green Apple","#4CC417"], ["223","LimeGreen (W3C)","#32CD32"], ["224","Pea Green","#52D017"], ["225","Kelly Green","#4CC552"], ["226","Zombie Green","#54C571"], ["227","Green Peas","#89C35C"], ["228","Dollar Bill Green","#85BB65"], ["229","Frog Green","#99C68E"], ["230","Turquoise Green","#A0D6B4"], ["231","DarkSeaGreen (W3C)","#8FBC8F"], ["232","Basil Green","#829F82"], ["233","Gray Green","#A2AD9C"], ["234","Iguana Green","#9CB071"], ["235","Citron Green","#8FB31D"], ["236","Acid Green","#B0BF1A"], ["237","Avocado Green","#B2C248"], ["238","Pistachio Green","#9DC209"], ["239","Salad Green","#A1C935"], ["240","YellowGreen (W3C)","#9ACD32"], ["241","Pastel Green","#77DD77"], ["242","Hummingbird Green","#7FE817"], ["243","Nebula Green","#59E817"], ["244","Stoplight Go Green","#57E964"], ["245","Neon Green","#16F529"], ["246","Jade Green","#5EFB6E"], ["247","Lime Mint Green","#36F57F"], ["248","SpringGreen (W3C)","#00FF7F"], ["249","MediumSpringGreen (W3C)","#00FA9A"], ["250","Emerald Green","#5FFB17"], ["251","Lime (W3C)","#00FF00"], ["252","LawnGreen (W3C)","#7CFC00"], ["253","Bright Green","#66FF00"], ["254","Chartreuse (W3C)","#7FFF00"], ["255","Yellow Lawn Green","#87F717"], ["256","Aloe Vera Green","#98F516"], ["257","Dull Green Yellow","#B1FB17"], ["258","Lemon Green","#ADF802"], ["259","GreenYellow (W3C)","#ADFF2F"], ["260","Chameleon Green","#BDF516"], ["261","Neon Yellow Green","#DAEE01"], ["262","Yellow Green Grosbeak","#E2F516"], ["263","Tea Green","#CCFB5D"], ["264","Slime Green","#BCE954"], ["265","Algae Green","#64E986"], ["266","LightGreen (W3C)","#90EE90"], ["267","Dragon Green","#6AFB92"], ["268","PaleGreen (W3C)","#98FB98"], ["269","Mint Green","#98FF98"], ["270","Green Thumb","#B5EAAA"], ["271","Organic Brown","#E3F9A6"], ["272","Light Jade","#C3FDB8"], ["273","Light Mint Green","#C2E5D3"], ["274","Light Rose Green","#DBF9DB"], ["275","Chrome White","#E8F1D4"], ["276","HoneyDew (W3C)","#F0FFF0"], ["277","MintCream (W3C)","#F5FFFA"], ["278","LemonChiffon (W3C)","#FFFACD"], ["279","Parchment","#FFFFC2"], ["280","Cream","#FFFFCC"], ["281","Cream White","#FFFDD0"], ["282","LightGoldenRodYellow (W3C)","#FAFAD2"], ["283","LightYellow (W3C)","#FFFFE0"], ["284","Beige (W3C)","#F5F5DC"], ["285","Cornsilk (W3C)","#FFF8DC"], ["286","Blonde","#FBF6D9"], ["287","Champagne","#F7E7CE"], ["288","AntiqueWhite (W3C)","#FAEBD7"], ["289","PapayaWhip (W3C)","#FFEFD5"], ["290","BlanchedAlmond (W3C)","#FFEBCD"], ["291","Bisque (W3C)","#FFE4C4"], ["292","Wheat (W3C)","#F5DEB3"], ["293","Moccasin (W3C)","#FFE4B5"], ["294","Peach","#FFE5B4"], ["295","Light Orange","#FED8B1"], ["296","PeachPuff (W3C)","#FFDAB9"], ["297","Coral Peach","#FBD5AB"], ["298","NavajoWhite (W3C)","#FFDEAD"], ["299","Golden Blonde","#FBE7A1"], ["300","Golden Silk","#F3E3C3"], ["301","Dark Blonde","#F0E2B6"], ["302","Light Gold","#F1E5AC"], ["303","Vanilla","#F3E5AB"], ["304","Tan Brown","#ECE5B6"], ["305","Dirty White","#E8E4C9"], ["306","PaleGoldenRod (W3C)","#EEE8AA"], ["307","Khaki (W3C)","#F0E68C"], ["308","Cardboard Brown","#EDDA74"], ["309","Harvest Gold","#EDE275"], ["310","Sun Yellow","#FFE87C"], ["311","Corn Yellow","#FFF380"], ["312","Pastel Yellow","#FAF884"], ["313","Neon Yellow","#FFFF33"], ["314","Yellow (W3C)","#FFFF00"], ["315","Canary Yellow","#FFEF00"], ["316","Banana Yellow","#F5E216"], ["317","Mustard Yellow","#FFDB58"], ["318","Golden Yellow","#FFDF00"], ["319","Bold Yellow","#F9DB24"], ["320","Rubber Ducky Yellow","#FFD801"], ["321","Gold (W3C)","#FFD700"], ["322","Bright Gold","#FDD017"], ["323","Chrome Gold","#FFCE44"], ["324","Golden Brown","#EAC117"], ["325","Deep Yellow","#F6BE00"], ["326","Macaroni and Cheese","#F2BB66"], ["327","Saffron","#FBB917"], ["328","Neon Gold","#FDBD01"], ["329","Beer","#FBB117"], ["330","Yellow Orange or Orange Yellow","#FFAE42"], ["331","Cantaloupe","#FFA62F"], ["332","Cheese Orange","#FFA600"], ["333","Orange (W3C)","#FFA500"], ["334","Brown Sand","#EE9A4D"], ["335","SandyBrown (W3C)","#F4A460"], ["336","Brown Sugar","#E2A76F"], ["337","Camel Brown","#C19A6B"], ["338","Deer Brown","#E6BF83"], ["339","BurlyWood (W3C)","#DEB887"], ["340","Tan (W3C)","#D2B48C"], ["341","Light French Beige","#C8AD7F"], ["342","Sand","#C2B280"], ["343","Sage","#BCB88A"], ["344","Fall Leaf Brown","#C8B560"], ["345","Ginger Brown","#C9BE62"], ["346","Bronze Gold","#C9AE5D"], ["347","DarkKhaki (W3C)","#BDB76B"], ["348","Olive Green","#BAB86C"], ["349","Brass","#B5A642"], ["350","Cookie Brown","#C7A317"], ["351","Metallic Gold","#D4AF37"], ["352","Bee Yellow","#E9AB17"], ["353","School Bus Yellow","#E8A317"], ["354","GoldenRod (W3C)","#DAA520"], ["355","Orange Gold","#D4A017"], ["356","Caramel","#C68E17"], ["357","DarkGoldenRod (W3C)","#B8860B"], ["358","Cinnamon","#C58917"], ["359","Peru (W3C)","#CD853F"], ["360","Bronze","#CD7F32"], ["361","Tiger Orange","#C88141"], ["362","Copper","#B87333"], ["363","Dark Gold","#AA6C39"], ["364","Metallic Bronze","#A97142"], ["365","Dark Almond","#AB784E"], ["366","Wood","#966F33"], ["367","Oak Brown","#806517"], ["368","Antique Bronze","#665D1E"], ["369","Hazel","#8E7618"], ["370","Dark Yellow","#8B8000"], ["371","Dark Moccasin","#827839"], ["372","Khaki Green","#8A865D"], ["373","Millennium Jade","#93917C"], ["374","Dark Beige","#9F8C76"], ["375","Bullet Shell","#AF9B60"], ["376","Army Brown","#827B60"], ["377","Sandstone","#786D5F"], ["378","Taupe","#483C32"], ["379","Mocha","#493D26"], ["380","Milk Chocolate","#513B1C"], ["381","Gray Brown","#3D3635"], ["382","Dark Coffee","#3B2F2F"], ["383","Old Burgundy","#43302E"], ["384","Western Charcoal","#49413F"], ["385","Bakers Brown","#5C3317"], ["386","Dark Brown","#654321"], ["387","Sepia Brown","#704214"], ["388","Dark Bronze","#804A00"], ["389","Coffee","#6F4E37"], ["390","Brown Bear","#835C3B"], ["391","Red Dirt","#7F5217"], ["392","Sepia","#7F462C"], ["393","Sienna (W3C)","#A0522D"], ["394","SaddleBrown (W3C)","#8B4513"], ["395","Dark Sienna","#8A4117"], ["396","Sangria","#7E3817"], ["397","Blood Red","#7E3517"], ["398","Chestnut","#954535"], ["399","Coral Brown","#9E4638"], ["400","Chestnut Red","#C34A2C"], ["401","Mahogany","#C04000"], ["402","Red Gold","#EB5406"], ["403","Red Fox","#C35817"], ["404","Dark Bisque","#B86500"], ["405","Light Brown","#B5651D"], ["406","Petra Gold","#B76734"], ["407","Rust","#C36241"], ["408","Copper Red","#CB6D51"], ["409","Orange Salmon","#C47451"], ["410","Chocolate (W3C)","#D2691E"], ["411","Sedona","#CC6600"], ["412","Papaya Orange","#E56717"], ["413","Halloween Orange","#E66C2C"], ["414","Neon Orange","#FF6700"], ["415","Bright Orange","#FF5F1F"], ["416","Pumpkin Orange","#F87217"], ["417","Carrot Orange","#F88017"], ["418","DarkOrange (W3C)","#FF8C00"], ["419","Construction Cone Orange","#F87431"], ["420","Indian Saffron","#FF7722"], ["421","Sunrise Orange","#E67451"], ["422","Mango Orange","#FF8040"], ["423","Coral (W3C)","#FF7F50"], ["424","Basket Ball Orange","#F88158"], ["425","Light Salmon Rose","#F9966B"], ["426","LightSalmon (W3C)","#FFA07A"], ["427","DarkSalmon (W3C)","#E9967A"], ["428","Tangerine","#E78A61"], ["429","Light Copper","#DA8A67"], ["430","Salmon Pink","#FF8674"], ["431","Salmon (W3C)","#FA8072"], ["432","Peach Pink","#F98B88"], ["433","LightCoral (W3C)","#F08080"], ["434","Pastel Red","#F67280"], ["435","Pink Coral","#E77471"], ["436","Bean Red","#F75D59"], ["437","Valentine Red","#E55451"], ["438","IndianRed (W3C)","#CD5C5C"], ["439","Tomato (W3C)","#FF6347"], ["440","Shocking Orange","#E55B3C"], ["441","OrangeRed (W3C)","#FF4500"], ["442","Red (W3C)","#FF0000"], ["443","Neon Red","#FD1C03"], ["444","Scarlet Red","#FF2400"], ["445","Ruby Red","#F62217"], ["446","Ferrari Red","#F70D1A"], ["447","Fire Engine Red","#F62817"], ["448","Lava Red","#E42217"], ["449","Love Red","#E41B17"], ["450","Grapefruit","#DC381F"], ["451","Cherry Red","#C24641"], ["452","Chilli Pepper","#C11B17"], ["453","FireBrick (W3C)","#B22222"], ["454","Tomato Sauce Red","#B21807"], ["455","Brown (W3C)","#A52A2A"], ["456","Carbon Red","#A70D2A"], ["457","Cranberry","#9F000F"], ["458","Saffron Red","#931314"], ["459","Crimson Red","#990000"], ["460","Red Wine or Wine Red","#990012"], ["461","DarkRed (W3C)","#8B0000"], ["462","Maroon (W3C)","#800000"], ["463","Burgundy","#8C001A"], ["464","Vermilion","#7E191B"], ["465","Deep Red","#800517"], ["466","Red Blood","#660000"], ["467","Blood Night","#551606"], ["468","Dark Scarlet","#560319"], ["469","Black Bean","#3D0C02"], ["470","Chocolate Brown","#3F000F"], ["471","Midnight","#2B1B17"], ["472","Purple Lily","#550A35"], ["473","Purple Maroon","#810541"], ["474","Plum Pie","#7D0541"], ["475","Plum Velvet","#7D0552"], ["476","Dark Raspberry","#872657"], ["477","Velvet Maroon","#7E354D"], ["478","Rosy-Finch","#7F4E52"], ["479","Dull Purple","#7F525D"], ["480","Puce","#7F5A58"], ["481","Rose Dust","#997070"], ["482","Pastel Brown","#B1907F"], ["483","Rosy Pink","#B38481"], ["484","RosyBrown (W3C)","#BC8F8F"], ["485","Khaki Rose","#C5908E"], ["486","Lipstick Pink","#C48793"], ["487","Pink Brown","#C48189"], ["488","Old Rose","#C08081"], ["489","Dusty Pink","#D58A94"], ["490","Pink Daisy","#E799A3"], ["491","Rose","#E8ADAA"], ["492","Dusty Rose","#C9A9A6"], ["493","Silver Pink","#C4AEAD"], ["494","Gold Pink","#E6C7C2"], ["495","Rose Gold","#ECC5C0"], ["496","Deep Peach","#FFCBA4"], ["497","Pastel Orange","#F8B88B"], ["498","Desert Sand","#EDC9AF"], ["499","Unbleached Silk","#FFDDCA"], ["500","Pig Pink","#FDD7E4"], ["501","Pale Pink","#F2D4D7"], ["502","Blush","#FFE6E8"], ["503","MistyRose (W3C)","#FFE4E1"], ["504","Pink Bubble Gum","#FFDFDD"], ["505","Light Rose","#FBCFCD"], ["506","Light Red","#FFCCCB"], ["507","Warm Pink","#F6C6BD"], ["508","Deep Rose","#FBBBB9"], ["509","Pink (W3C)","#FFC0CB"], ["510","LightPink (W3C)","#FFB6C1"], ["511","Soft Pink","#FFB8BF"], ["512","Donut Pink","#FAAFBE"], ["513","Baby Pink","#FAAFBA"], ["514","Flamingo Pink","#F9A7B0"], ["515","Pastel Pink","#FEA3AA"], ["516","Rose Pink or Pink Rose","#E7A1B0"], ["517","Cadillac Pink","#E38AAE"], ["518","Carnation Pink","#F778A1"], ["519","Pastel Rose","#E5788F"], ["520","Blush Red","#E56E94"], ["521","PaleVioletRed (W3C)","#DB7093"], ["522","Purple Pink","#D16587"], ["523","Tulip Pink","#C25A7C"], ["524","Bashful Pink","#C25283"], ["525","Dark Pink","#E75480"], ["526","Dark Hot Pink","#F660AB"], ["527","HotPink (W3C)","#FF69B4"], ["528","Watermelon Pink","#FC6C85"], ["529","Violet Red","#F6358A"], ["530","Hot Deep Pink","#F52887"], ["531","Bright Pink","#FF007F"], ["532","DeepPink (W3C)","#FF1493"], ["533","Neon Pink","#F535AA"], ["534","Chrome Pink","#FF33AA"], ["535","Neon Hot Pink","#FD349C"], ["536","Pink Cupcake","#E45E9D"], ["537","Royal Pink","#E759AC"], ["538","Dimorphotheca Magenta","#E3319D"], ["539","Pink Lemonade","#E4287C"], ["540","Red Pink","#FA2A55"], ["541","Raspberry","#E30B5D"], ["542","Crimson (W3C)","#DC143C"], ["543","Bright Maroon","#C32148"], ["544","Rose Red","#C21E56"], ["545","Rogue Pink","#C12869"], ["546","Burnt Pink","#C12267"], ["547","Pink Violet","#CA226B"], ["548","Magenta Pink","#CC338B"], ["549","MediumVioletRed (W3C)","#C71585"], ["550","Dark Carnation Pink","#C12283"], ["551","Raspberry Purple","#B3446C"], ["552","Pink Plum","#B93B8F"], ["553","Orchid (W3C)","#DA70D6"], ["554","Deep Mauve","#DF73D4"], ["555","Violet (W3C)","#EE82EE"], ["556","Fuchsia Pink","#FF77FF"], ["557","Bright Neon Pink","#F433FF"], ["558","Fuchsia or Magenta (W3C)","#FF00FF"], ["559","Crimson Purple","#E238EC"], ["560","Heliotrope Purple","#D462FF"], ["561","Tyrian Purple","#C45AEC"], ["562","MediumOrchid (W3C)","#BA55D3"], ["563","Purple Flower","#A74AC7"], ["564","Orchid Purple","#B048B5"], ["565","Rich Lilac","#B666D2"], ["566","Pastel Violet","#D291BC"], ["567","Mauve Taupe","#915F6D"], ["568","Viola Purple","#7E587E"], ["569","Eggplant","#614051"], ["570","Plum Purple","#583759"], ["571","Grape","#5E5A80"], ["572","Purple Navy","#4E5180"], ["573","SlateBlue (W3C)","#6A5ACD"], ["574","Blue Lotus","#6960EC"], ["575","Blurple","#5865F2"], ["576","Light Slate Blue","#736AFF"], ["577","MediumSlateBlue (W3C)","#7B68EE"], ["578","Periwinkle Purple","#7575CF"], ["579","Very Peri","#6667AB"], ["580","Bright Grape","#6F2DA8"], ["581","Purple Amethyst","#6C2DC7"], ["582","Bright Purple","#6A0DAD"], ["583","Deep Periwinkle","#5453A6"], ["584","DarkSlateBlue (W3C)","#483D8B"], ["585","Purple Haze","#4E387E"], ["586","Purple Iris","#571B7E"], ["587","Dark Purple","#4B0150"], ["588","Deep Purple","#36013F"], ["589","Midnight Purple","#2E1A47"], ["590","Purple Monster","#461B7E"], ["591","Indigo (W3C)","#4B0082"], ["592","Blue Whale","#342D7E"], ["593","RebeccaPurple (W3C)","#663399"], ["594","Purple Jam","#6A287E"], ["595","DarkMagenta (W3C)","#8B008B"], ["596","Purple (W3C)","#800080"], ["597","French Lilac","#86608E"], ["598","DarkOrchid (W3C)","#9932CC"], ["599","DarkViolet (W3C)","#9400D3"], ["600","Purple Violet","#8D38C9"], ["601","Jasmine Purple","#A23BEC"], ["602","Purple Daffodil","#B041FF"], ["603","Clematis Violet","#842DCE"], ["604","BlueViolet (W3C)","#8A2BE2"], ["605","Purple Sage Bush","#7A5DC7"], ["606","Lovely Purple","#7F38EC"], ["607","Neon Purple","#9D00FF"], ["608","Purple Plum","#8E35EF"], ["609","Aztech Purple","#893BFF"], ["610","MediumPurple (W3C)","#9370DB"], ["611","Light Purple","#8467D7"], ["612","Crocus Purple","#9172EC"], ["613","Purple Mimosa","#9E7BFF"], ["614","Periwinkle","#CCCCFF"], ["615","Pale Lilac","#DCD0FF"], ["616","Lavender Purple","#967BB6"], ["617","Rose Purple","#B09FCA"], ["618","Lilac","#C8A2C8"], ["619","Mauve","#E0B0FF"], ["620","Bright Lilac","#D891EF"], ["621","Purple Dragon","#C38EC7"], ["622","Plum (W3C)","#DDA0DD"], ["623","Blush Pink","#E6A9EC"], ["624","Pastel Purple","#F2A2E8"], ["625","Blossom Pink","#F9B7FF"], ["626","Wisteria Purple","#C6AEC7"], ["627","Purple Thistle","#D2B9D3"], ["628","Thistle (W3C)","#D8BFD8"], ["629","Purple White","#DFD3E3"], ["630","Periwinkle Pink","#E9CFEC"], ["631","Cotton Candy","#FCDFFF"], ["632","Lavender Pinocchio","#EBDDE2"], ["633","Dark White","#E1D9D1"], ["634","Ash White","#E9E4D4"], ["635","White Chocolate","#EDE6D6"], ["636","Soft Ivory","#FAF0DD"], ["637","Off White","#F8F0E3"], ["638","Pearl White","#F8F6F0"], ["639","Red White","#F3E8EA"], ["640","LavenderBlush (W3C)","#FFF0F5"], ["641","Pearl","#FDEEF4"], ["642","Egg Shell","#FFF9E3"], ["643","OldLace (W3C)","#FEF0E3"], ["644","Linen (W3C)","#FAF0E6"], ["645","SeaShell (W3C)","#FFF5EE"], ["646","Bone White","#F9F6EE"], ["647","Rice","#FAF5EF"], ["648","FloralWhite (W3C)","#FFFAF0"], ["649","Ivory (W3C)","#FFFFF0"], ["650","White Gold","#FFFFF4"], ["651","Light White","#FFFFF7"], ["652","WhiteSmoke (W3C)","#F5F5F5"], ["653","Cotton","#FBFBF9"], ["654","Snow (W3C)","#FFFAFA"], ["655","Milk White","#FEFCFF"], ["656","Half White","#FFFEFA"], ["657","White (W3C)","#FFFFFF"], ];
_________________ Patrice
|
|